问题 A: C++综合练习——MyArray类
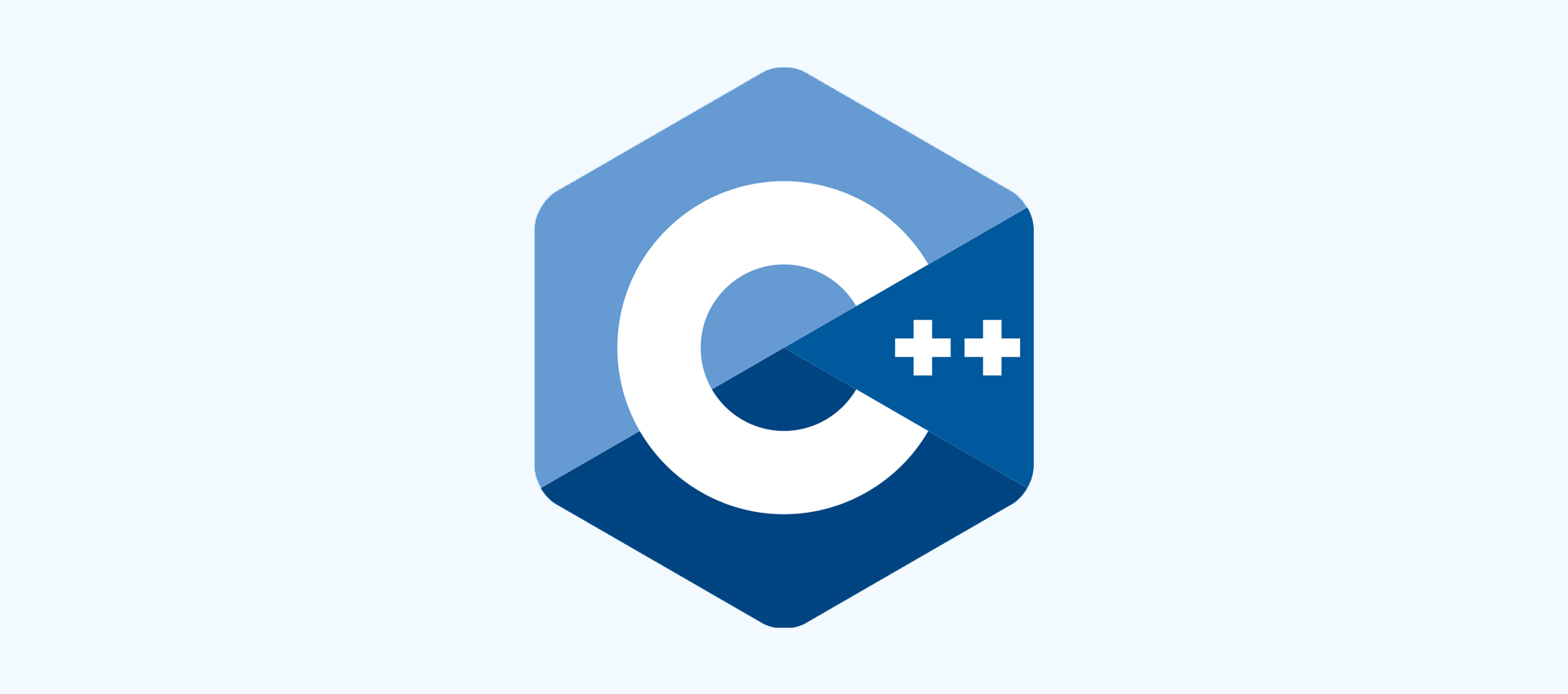
问题 A: C++综合练习——MyArray类
Ramsayi题目描述
设计一个 MyArray 类,用来模拟一个由小到大的有序的整数序列,实现输入一个整数,将其插入序列,并保持该序列由小到大有序。MyArray 类原型如下:
class MyArray
{private:
int a[50]; //存储一个由小到大有序的整数序列
int n; //记录序列中数据的个数
public:
MyArray(int x) { n=x; } //初始化,通过 x 的值确定序列的大小
void input(); //从键盘输入 n 个由小到大有序的整数
void insert(int k); //将 k 值按由小到大的顺序插入到序列中(注意,插入一个数据后,n 值要增一)
void output(); //输出序列中的数据
};
输入
第一行有一个正整数 n,表示原始的整数序列长度为 n,保证 n 不超过 50。
第二行有 n 个整数,表示原始的整数序列,保证这个序列是从小到大给出的。
第三行有一个整数,表示需要插入的整数。
输出
在一行中输出 n+1 个整数,表示完成插入的整数序列。每个整数后输出一个空格。请注意行尾输出换行。
样例输入
1 | 10 |
样例输出
1 | 1 2 3 4 5 6 7 8 9 10 11 |
提示
1 |
|
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果